The SOLID principles are a set of five fundamental design guidelines in object-oriented programming that help developers create more maintainable, understandable, and flexible software. Coined by Robert C. Martin, also known as “Uncle Bob,” these principles are essential for anyone looking to write clean, efficient, and scalable code. Let’s explore each of these principles in detail.
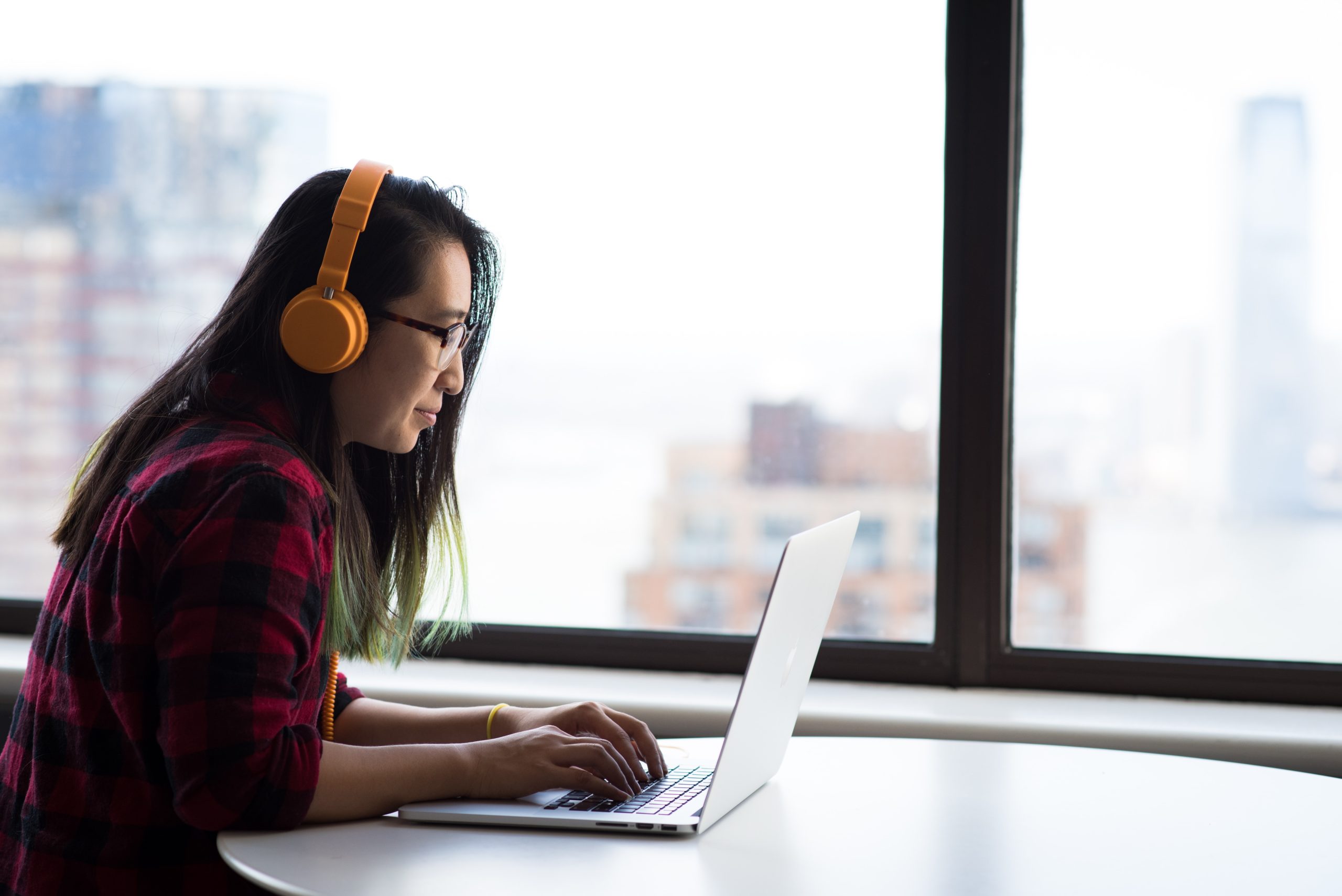
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should have only one job or responsibility. This principle emphasizes the idea that each class should focus on a single task or function, which makes the code easier to understand, maintain, and modify.
For example, consider a class that handles both user authentication and data logging. According to SRP, these two responsibilities should be separated into different classes. This separation allows you to make changes to one responsibility without affecting the other, thereby reducing the risk of bugs and making the system more robust.
2. Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that the behavior of a class can be extended without modifying its existing code.
To adhere to this principle, developers often use inheritance and interfaces. For example, if you have a class that calculates the area of different shapes, you can extend its functionality by adding new shapes without modifying the existing class code. This approach prevents bugs from being introduced into existing code and makes the system more scalable.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle, introduced by Barbara Liskov, states that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. In simpler terms, a subclass should be able to perform the same functions as its superclass without causing errors.
This principle ensures that the inheritance hierarchy is used properly, and it promotes the use of polymorphism. For example, if you have a base class `Bird` with a method `fly()`, all subclasses like `Sparrow` or `Eagle` should be able to use the `fly()` method in a way that makes sense within the program context. If a subclass like `Penguin` does not adhere to this, it indicates a design flaw.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle suggests that a client should not be forced to implement interfaces it does not use. Instead of having a large, monolithic interface, it’s better to create smaller, more specific interfaces so that classes can implement only the methods they need.
For example, instead of having a large interface `Animal` with methods like `fly()`, `swim()`, and `walk()`, it’s better to break it down into smaller interfaces like `FlyingAnimal`, `SwimmingAnimal`, and `WalkingAnimal`. This way, a class that implements the `FlyingAnimal` interface won’t be forced to implement unnecessary methods like `swim()`.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle advocates that high-level modules should not depend on low-level modules but rather on abstractions. This means that both high-level and low-level modules should depend on interfaces or abstract classes rather than concrete implementations.
By adhering to DIP, developers can create more flexible and reusable code. For instance, instead of a class depending directly on a concrete implementation of a database connection, it should depend on an interface that defines the connection methods. This allows you to swap out different database implementations without changing the code that depends on the interface.
The SOLID principles are essential for writing clean, maintainable, and scalable object-oriented code. By adhering to these principles, developers can avoid common pitfalls and design flaws, making their software more robust and easier to manage over time. Whether you’re a beginner or an experienced developer, integrating SOLID principles into your design process will significantly enhance the quality of your codebase.