Anchor links are commonly used in web development to jump to specific sections within a webpage. However, when combined with an accordion—a UI pattern that displays collapsible content panels—anchor links can offer an interactive and dynamic user experience. By coding an anchor link that opens an accordion panel upon being clicked, you can direct users to specific content within a collapsed section, making navigation more intuitive. Here’s how to code an anchor link that not only jumps to an accordion section but also opens it automatically.
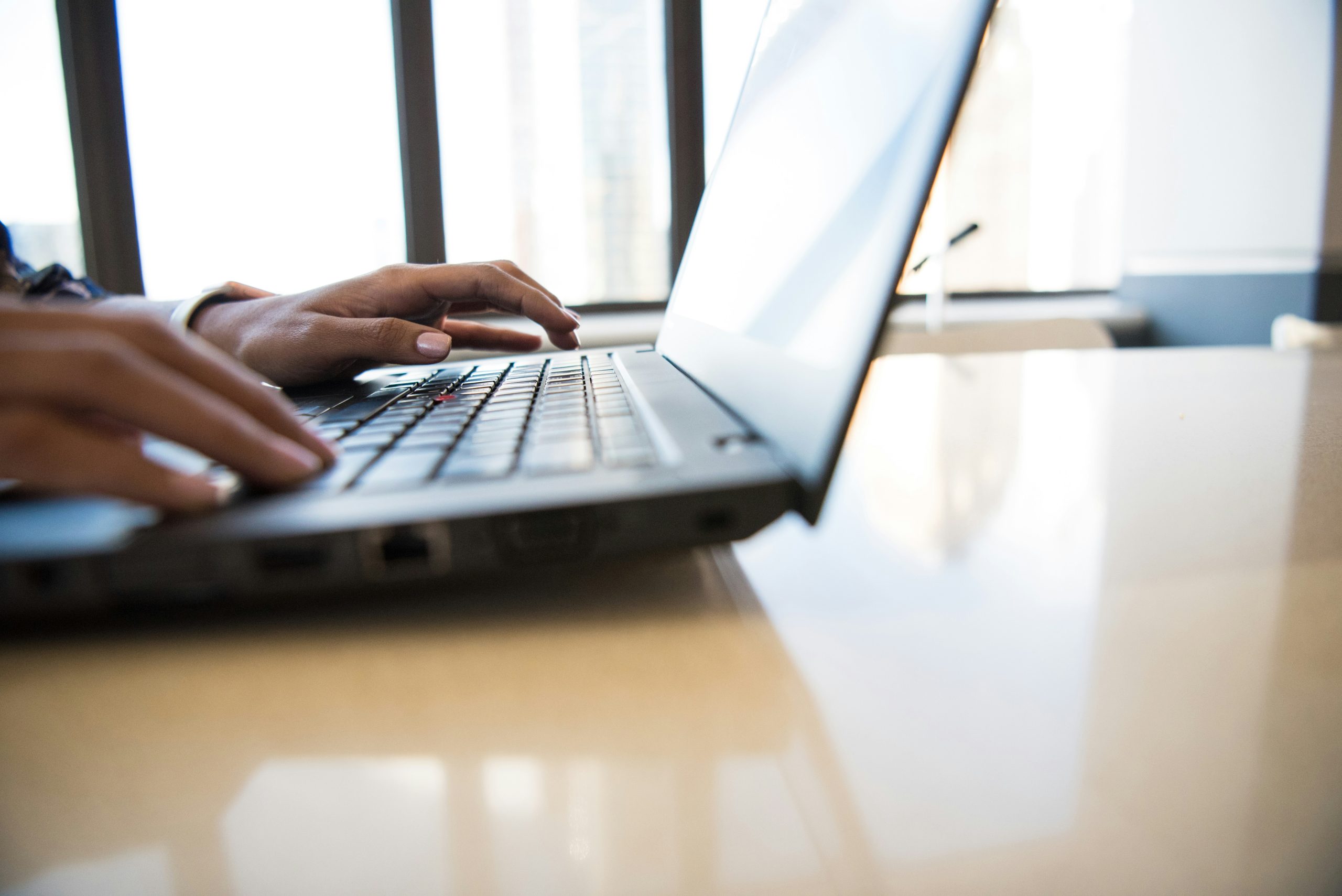
Step 1: Set Up the HTML Structure
First, we need a basic HTML structure for the accordion. An accordion typically consists of multiple collapsible panels, each with a header (to trigger the collapse) and a content area that displays or hides content.
Here’s a simple example:
“`html
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>Accordion with Anchor Link</title>
<link rel=”stylesheet” href=”styles.css”>
</head>
<body>
<a href=”section1″ class=”accordion-link”>Go to Section 1</a>
<a href=”section2″ class=”accordion-link”>Go to Section 2</a>
<div class=”accordion”>
<div class=”accordion-item” id=”section1″>
<button class=”accordion-header” onclick=”toggleAccordion(‘section1’)”>Section 1</button>
<div class=”accordion-content”>
<p>This is the content for Section 1.</p>
</div>
</div>
<div class=”accordion-item” id=”section2″>
<button class=”accordion-header” onclick=”toggleAccordion(‘section2’)”>Section 2</button>
<div class=”accordion-content”>
<p>This is the content for Section 2.</p>
</div>
</div>
</div>
<script src=”script.js”></script>
</body>
</html>
“`
In this example:
– The links (`<a href=”section1″>Go to Section 1</a>`) navigate to specific accordion sections.
– Each accordion section has a unique `id` attribute (`id=”section1″`), which allows the anchor link to target it.
Step 2: Style the Accordion
In `styles.css`, add some basic styling for the accordion. This will include hiding the content by default and adding some transition effects.
“`css
.accordion {
margin-top: 20px;
}
.accordion-item {
border: 1px solid ccc;
margin-bottom: 5px;
}
.accordion-header {
background-color: f0f0f0;
cursor: pointer;
padding: 10px;
width: 100%;
text-align: left;
border: none;
}
.accordion-content {
display: none;
padding: 10px;
border-top: 1px solid ccc;
}
.accordion-content.active {
display: block;
}
“`
Step 3: Create JavaScript to Toggle Accordion Panels
To control the accordion behavior, we’ll add a JavaScript function in `script.js` that toggles the visibility of each accordion section. This function will add or remove the `active` class from the `accordion-content` to show or hide it.
“`javascript
function toggleAccordion(sectionId) {
const content = document.querySelector(`${sectionId} .accordion-content`);
content.classList.toggle(‘active’);
}
“`
This function checks the targeted section’s content area (with `sectionId` passed as a parameter) and toggles the `active` class, controlling the `display` property set in the CSS.
Step 4: Open the Accordion Section Using Anchor Links
Now, we need to make sure that clicking an anchor link not only scrolls to the section but also opens it. We can achieve this by listening for the `hashchange` event, which detects when the URL hash changes (e.g., clicking `section1`). When the hash changes, we’ll automatically trigger the `toggleAccordion` function.
Add the following code to `script.js`:
“`javascript
window.addEventListener(‘hashchange’, () => {
const sectionId = window.location.hash.substring(1);
if (sectionId) {
const content = document.querySelector(`${sectionId} .accordion-content`);
if (content && !content.classList.contains(‘active’)) {
toggleAccordion(sectionId);
}
// Scroll smoothly to the section
document.getElementById(sectionId).scrollIntoView({ behavior: ‘smooth’ });
}
});
“`
This event listener does the following:
1. Checks if there’s a new `hash` in the URL (i.e., `section1` or `section2`).
2. Retrieves the section `id` from the URL hash.
3. Toggles the accordion section open if it’s not already open.
4. Smoothly scrolls to the section.
Step 5: Initial Load Check (Optional)
If a user directly lands on a URL with a hash (e.g., `yourpage.htmlsection1`), you might want to open the accordion panel automatically. Add this code at the end of `script.js`:
“`javascript
document.addEventListener(‘DOMContentLoaded’, () => {
const sectionId = window.location.hash.substring(1);
if (sectionId) {
toggleAccordion(sectionId);
document.getElementById(sectionId).scrollIntoView({ behavior: ‘smooth’ });
}
});
“`
By combining HTML, CSS, and JavaScript, you can create anchor links that open accordion sections dynamically. This setup makes it easy for users to navigate directly to specific content in collapsed sections, improving their experience on your website.