Developers building web applications often look for ways to integrate payment processing platforms like Stripe into their apps. Dash, a Python framework developed by Plotly, is primarily used for creating interactive data visualizations and dashboards. But can Stripe, a widely popular payment gateway, be added to a Dash app? The answer is yes, and this article will explain how you can integrate these two tools effectively.
Dash is built on Flask, React, and Plotly, making it highly customizable. While it does not come with built-in payment integration tools like Stripe, its Flask backbone allows developers to use Stripe’s Python SDK or JavaScript API for embedding payment functionality. This opens up exciting possibilities for developers aiming to create data-rich, interactive applications with monetized features.
Why Combine Stripe and Dash?
Stripe is highly regarded for its ease of use, security, and support for multiple payment methods, including credit cards, wallets, and global currency processing. By incorporating Stripe into a Dash app, developers can:
- Monetize data-driven services: Charge users for accessing dashboards or premium datasets.
- Offer subscription models: Implement recurring payments for ongoing access to content or services.
- Facilitate one-time payments: Handle transactional payments for digital goods directly within the app.
With the right setup, Stripe enables secure and seamless transactions, complementing Dash’s high-performance capabilities to deliver a robust experience to users.
Steps to Adding Stripe to Your Dash App
Here’s an outline of the process to integrate Stripe into a Dash app:
1. Set Up a Stripe Account
Before writing any code, the first step is to create a Stripe account and set up a new project in the Stripe Dashboard. This provides the API keys required to interact with Stripe’s payment gateway. Ensure you have access to both testing and live keys for development and production environments.
2. Install Required Libraries
Dash apps are Python-based, so you’ll need the Stripe Python SDK for server-side interactions. You can install it with the following command:
pip install stripe
For client-side payment handling, Stripe provides a JavaScript library called Stripe.js. This library is particularly useful for securely collecting and tokenizing card details from users.
3. Build the Payment Interface
Create an interface in your Dash app to allow users to make payments. This could be a simple form asking for billing details and the amount to be charged. Since Dash uses React for rendering HTML, you can write custom callback functions to dynamically display components of the payment process, such as success or failure messages.
4. Implement Server-Side Logic
Dash runs on Flask, making it possible to define server routes to handle payment processing. In your Dash app’s `app.server` object, you can implement routes where Stripe’s API processes requests and manages payment intents. Here’s an example of handling a simple Stripe payment on the server:
import stripe
stripe.api_key = 'your_secret_key'
@app.server.route('/create-payment-intent', methods=['POST'])
def create_payment():
try:
# Create a payment intent
payment_intent = stripe.PaymentIntent.create(
amount=1000, # Amount in cents
currency='usd',
)
return jsonify({'clientSecret': payment_intent['client_secret']})
except Exception as e:
return jsonify({'error': str(e)})
You can then use this server-side route to create a payment flow, further linking it with Stripe.js on the client side.
5. Secure Your Application
Payment processing involves sensitive user data. Always use secure HTTPS protocols and keep your API keys confidential. For live environments, leverage Stripe’s built-in tools such as fraud detection and PCI compliance automation.
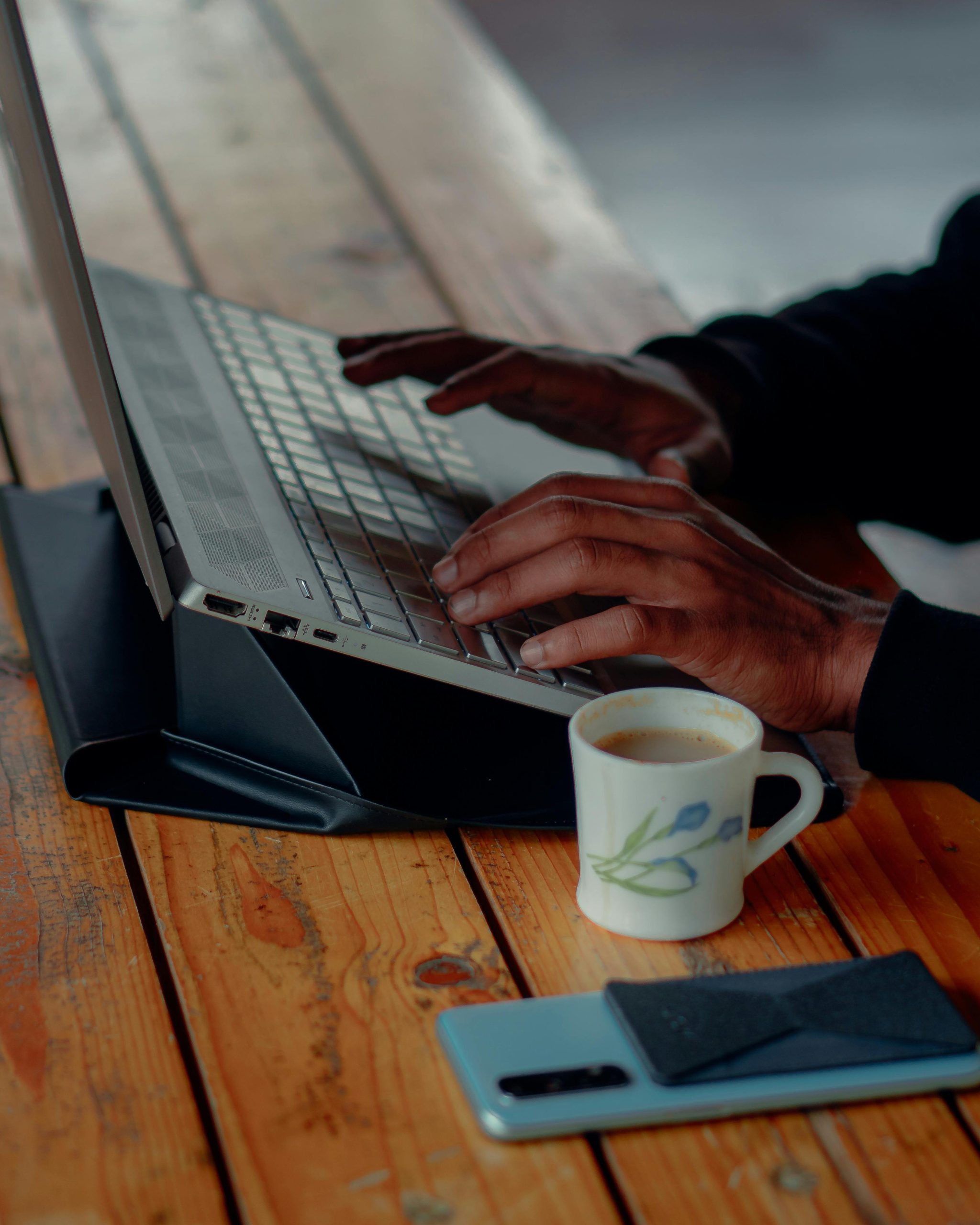
Challenges You Might Face
Although integrating Stripe with Dash is straightforward, there may be challenges:
- Asynchronous callbacks: Managing server-side logic in Dash requires careful planning, especially for real-time feedback on payments.
- Styling issues: Dash’s simplicity can sometimes make it harder to implement custom UI elements from Stripe.js smoothly.
- Debugging errors: Stripe’s extensive documentation helps a lot, but minor configuration issues can still lead to confusion.
Investing time in testing your integration thoroughly can minimize these hurdles.
FAQs
- Can I use Stripe with Dash without Flask?
- No, Dash relies on Flask for its server-side operations. The integration is made possible through Flask’s backend.
- What payment methods does Stripe support?
- Stripe supports many methods, including credit/debit cards, ACH transfers, Apple Pay, Google Pay, and various local alternatives.
- Is adding Stripe to Dash suitable for large-scale applications?
- Yes, Stripe’s scalability and secure infrastructure make it suitable for handling payments in applications of all sizes.
- Are there alternatives to Stripe for integrating payments into Dash?
- Yes, platforms like PayPal, Braintree, or Square can also be integrated, but Stripe is among the most developer-friendly options.
With a bit of effort, adding Stripe to a Dash app can unlock significant value, enabling monetization while maintaining the interactive and data-rich focus of Dash applications.