Displaying Google Reviews on a WordPress website can increase trust and credibility, encouraging more visitors to engage with a business. While many plugins can achieve this, some users prefer a manual approach to reduce dependency on third-party tools and improve site performance.
Why Display Google Reviews Without a Plugin?
Using a plugin can simplify the process, but it may introduce several drawbacks:
- Plugins can slow down website performance.
- Some plugins require a premium subscription for full functionality.
- Manual integration provides complete control over the display and styling.
By manually embedding Google Reviews, users can have greater flexibility while maintaining a lightweight website.
Step 1: Obtain Google Places API Key
To retrieve and display Google Reviews, users need to generate a Google Places API key.
- Go to the Google Cloud Console and log in.
- Create a new project or select an existing one.
- Navigate to the “API & Services” section and enable the Places API.
- Go to “Credentials” and click on “Create Credentials” to generate an API key.
- Restrict the API key for security by limiting it to specific domains.
Once the key is generated, it will be used to retrieve business reviews from Google.
Step 2: Find the Place ID
Google assigns a unique Place ID to each business location. To retrieve it:
- Visit the Google Place ID Finder.
- Enter the business name or address.
- Copy the provided Place ID.
Step 3: Fetch Google Reviews Using JavaScript
To display the reviews dynamically, a custom script can be used.
<script>
function loadReviews() {
var placeId = 'YOUR_PLACE_ID';
var apiKey = 'YOUR_API_KEY';
var url = `https://maps.googleapis.com/maps/api/place/details/json?placeid=${placeId}&key=${apiKey}&fields=reviews`;
fetch(url)
.then(response => response.json())
.then(data => {
if (data.result.reviews) {
var reviewsContainer = document.getElementById('google-reviews');
data.result.reviews.forEach(review => {
var reviewElement = document.createElement('div');
reviewElement.innerHTML = `
<div class="review">
<h3>${review.author_name}</h3>
<p>${review.text}</p>
<strong>Rating:</strong> ${review.rating}/5
</div>
`;
reviewsContainer.appendChild(reviewElement);
});
}
})
.catch(err => console.error('Failed to fetch reviews', err));
}
window.onload = loadReviews;
</script>
Ensure to replace YOUR_PLACE_ID
and YOUR_API_KEY
with the actual values obtained in the previous steps.
Step 4: Display Reviews in WordPress
In the WordPress theme file where the reviews should appear (e.g., footer.php
or sidebar.php
), add the following HTML code:
<div id="google-reviews"></div>
This div will be dynamically populated with reviews fetched from Google. CSS can be applied to enhance styling.
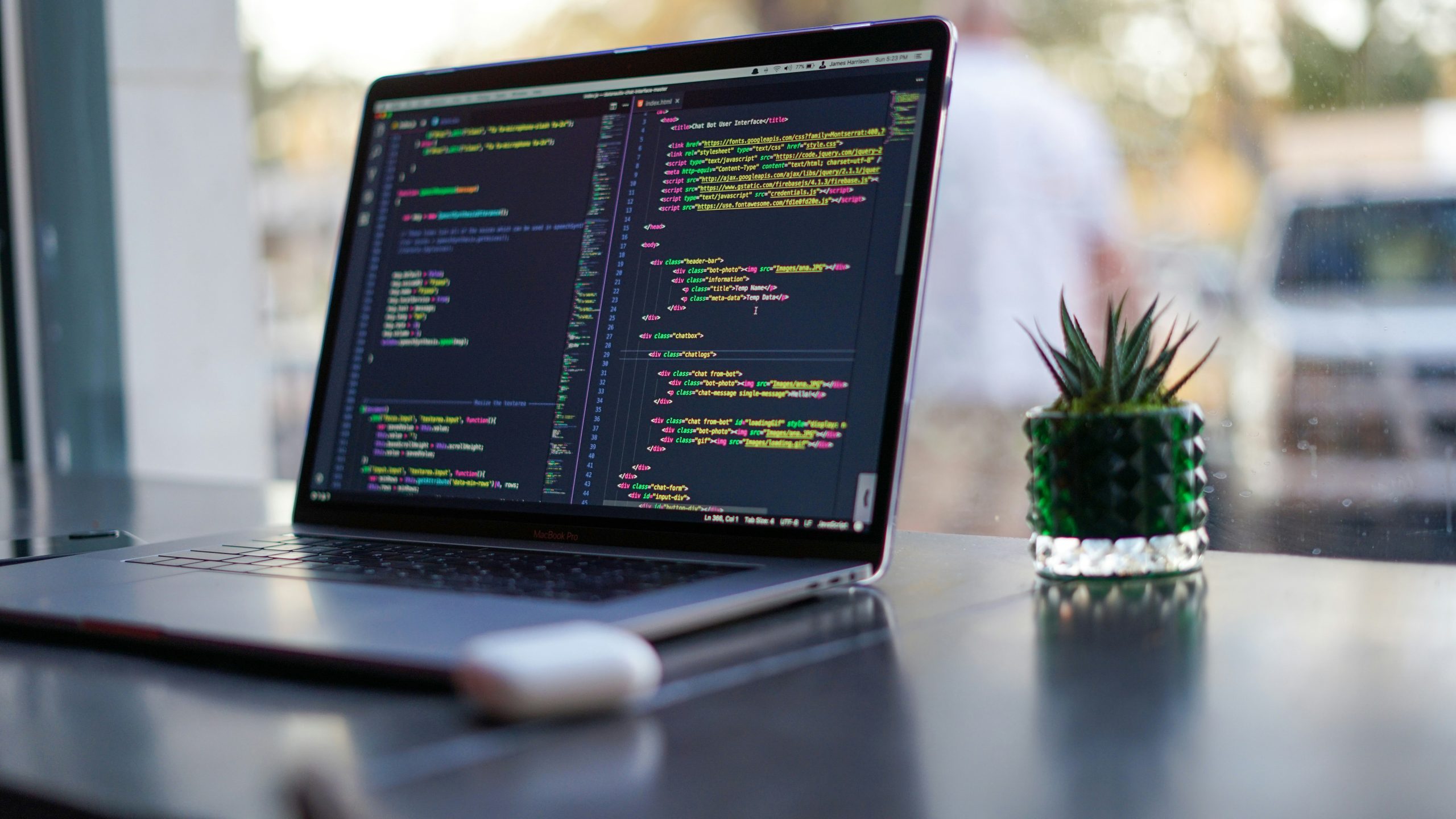
Step 5: Add Custom Styling
To make the displayed reviews visually appealing, custom CSS can be applied:
<style>
#google-reviews {
background-color: #f9f9f9;
padding: 20px;
border-radius: 5px;
max-width: 600px;
margin: auto;
}
.review {
border-bottom: 1px solid #ddd;
padding: 10px;
margin-bottom: 10px;
}
.review:last-child {
border-bottom: none;
}
</style>
Final Thoughts
Displaying Google Reviews in WordPress without a plugin can be an efficient and flexible way to enhance a website. Although it requires some coding knowledge, the approach minimizes reliance on external plugins, improving site speed and security.
Frequently Asked Questions
Can this method be used without an API key?
No, Google requires an API key to fetch business reviews securely.
How often are the reviews updated?
The JavaScript fetch function retrieves live data every time a visitor loads the page.
What if no reviews appear?
Check whether the Place ID and API Key are correct and ensure that the Places API is enabled in Google Cloud.
Can I filter the number of displayed reviews?
Yes, by modifying the JavaScript function, it is possible to limit the number of displayed reviews.
Does this method affect website performance?
Since the script runs on the client-side, it does not significantly impact page load speed.